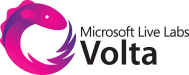
[C# Sources here]
In this post I’m going to show how to create a simple Volta application with a DragPanel-like object on the page. You can see how ASP.NET AJAX Toolkit DragPanel works here.
This is a very practical example, if you need explanations about Volta architecture and stuff like that; you better go to the Microsoft Live Labs Volta Website
First of all, you have to download and install Volta CTP, you can find it here.
Volta works only with Visual Studio 2008 RTM or Beta2
Step 1: Create Volta application
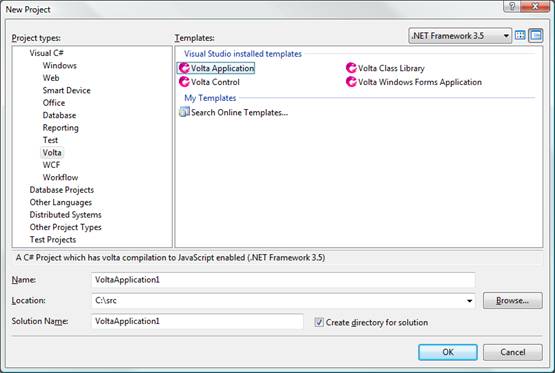
You have to create a new project, and then select “Volta Application”.
Step 2: Design User Interface
You have to design the user interface; you can use every HTML designer. In this case we will use Visual Studio HTML designer.
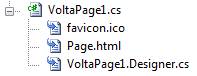
You have to modify Page.html.
Thanks to Volta, HTML interface is totally detached from the rest of the application. As we will see, the UI code will be standard HTML 4.01. with no tags or something like that.
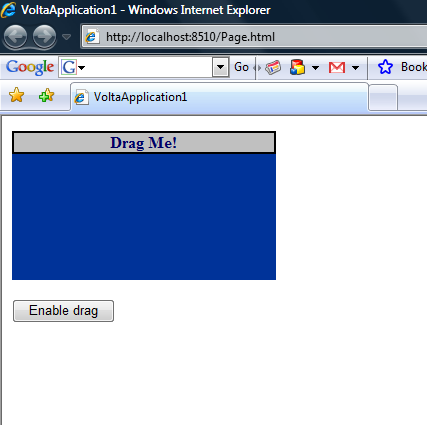
This is how we want to make our application looks like.
DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"
"http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>VoltaApplication1title>
<style type="text/css">
#DraggableDiv
{
width: 264px;
height: 149px;
position: relative;
background-color: #003399;
color: #FFFF00;
text-align: center;
}
#Handle
{
border-color: #000000;
background-color: #C0C0C0;
color: #000066;
text-align: center;
border-width: thin;
border-style: solid;
}
style>
head>
<body>
<div id="DraggableDiv">
<div id="Handle">
<b>Drag Me!b>
div>
div>
<p>
<button id="ButtonEnable">
Enable drag
button>
p>
body>
We have 2 “div” tags. The first is “DraggableDiv” which is the main container, the second is ”Handle” which is the handle used to drag “DraggableDiv”.
We also have a button: "ButtonEnable" which will be used to enable dragging capabilities on “DraggableDiv”.
Step 3:Import Javascript library
Dom-Drag.js
In this example I’m going to use DOM-DRAG, made by Aaron Boodman. You can find a guide about it here. Direct link to download it is here
The file is dom-drag.js.
To activate dragging capabilities we just have to call Drag.Init javascript function. Here there is an example of how to use it:
Drag.init(document.getElementById("Handle"),document.getElementById("DraggableDiv"));
The first parameter is a reference to the Handle div, the second one is a reference to the main container.
In this example we are going to call Drag.Init from C# code instead of Javascript code, to make this possible we have to make a “wrapper” C# class.
Adding dom.drag.js and modifying AssemblyInfo.cs
You have to add dom-drag.js to your project and then you have to add this line to your AssemblyInfo.cs:
[assembly: ScriptReference("dom-drag.js")]
Creating C# Wrapper Class
You have to add a simple C# class to your solution and to call it DomDrag
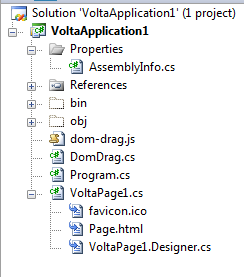
This is the code of the class
class DomDrag
{
[Import("Drag.init")]
public static extern void Init(HtmlElement handle, HtmlElement container);
With the “import” attribute you tell the Volta rewriter that Init method wraps “Drag.Init” Javascript function defined in dom-drag.js.
“Drag.Init” needs 2 main parameters (there are others available but in this case we are going to use just 2 of them): “handle” and “container” which are two references to our html div elements.
Step 4: Write the code behind UI
In the 4th step we are going to write the code behind “Page.html”, all this C# code will be compiled in MSIL by C# 3.0 compiler and this will be rewritten in Javascript code by Volta recompiler.
Here is the code of VoltaPage1.cs
public partial class VoltaPage1 : Page
{
Button butEnable;
HtmlElement dragDiv;
HtmlElement dragHandle;
public VoltaPage1()
{
InitializeComponent();
}
partial void InitializeComponent()
{
/*
Here we get the references to our
html controls using GetById generic methods
*/
butEnable = Document.GetById<Button>("ButtonEnable");
dragDiv = Document.GetById<Div>("DraggableDiv");
dragHandle = Document.GetById<Div>("Handle");
//We create our Click handler
butEnable.Click += ButtonEnable_Click;
}
void ButtonEnable_Click()
{
//Calls the init function
DomDrag.Init(dragHandle, dragDiv);
butEnable.Disabled = true;
}
Finally you have to manually copy dom-drag.js in "bin\Debug\Volta". I’m looking for a workaround but I haven’t found it yet.
Done!
Press F5 and enjoy your new Volta application.